I have looked into a number of ways to do this, but ended up going with a 3rd party tool that already provides 99% of the functionality. The only drawback was the fact that spaces default to
and Blogger randomly deletes these. If you'd like to start working on your own, you can start using the code snippet I have below. Unfortunately, this only highlights
C# Keywords and nothing else. You can also download the source code for
CopySourceAsHtml, which has a Rtf parsing approach.
Copy Source As Html Visual Studio Add-In
Download
CopySourceAsHtml Visual Studio Add-In. (As of this writing, only available for 2005).
Install the Add-In in Visual Studio. (I had to restart Visual Studio after installing, but this may have something to do with Windows Vista interoperability.)
Select some code and then pick "Copy as HTML" from the content menu item that appears. (Due to a bug in the Blogger html parser that randomly deletes spaces ( ), you will want to do steps 3-4. This will ensure that the tabs are preserved. Otherwise, you will find that tabs may disappear after you try to edit the post later on.)
When the "Copy as HTML" dialog appears, ensure that only the following checkboxes are selected: "Wrap Words", "Embed Styles", "Strip line breaks", "Remove indentation".
Paste the text into Notepad and then replace with <span style='margin-left:2em' />
Copy the results into Blogger.
If you want to add comments above or below, make sure you are in Edit Html mode and add text before or after the markup. You can then switch to Compose mode.
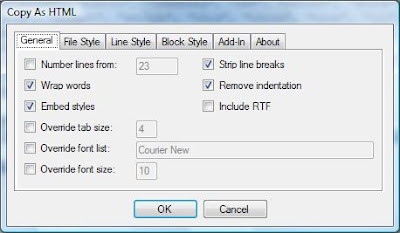
Create Your Own Parser
This is a very simple code snippet that could be used to start your own parser. It does create Blogger friendly tabs, but only highlights keywords. (Note: the following code was posted using the first approach.)
private string UpdateBloggerHTML(string plainToHtml)
{
string[] cSharpKeywords = {"using", "abstract", "as", "base", "bool", "break", "byte", "case", "catch", "char", "checked",
"class", "const", "continue", "decimal", "default", "delegate", "do", "double", "else", "enum", "event", "explicit",
"extern", "false", "finally", "fixed", "float", "for", "foreach", "goto", "if", "implicit", "in", "int", "interface",
"internal", "is", "lock", "long", "namespace", "new", "null", "object", "operator", "out", "override", "params",
"private", "protected", "public", "readonly", "ref", "return", "sbyte", "sealed", "short", "sizeof", "stackalloc",
"static", "string", "struct", "switch", "this", "throw", "true", "try", "typeof", "uint", "ulong", "unchecked",
"unsafe", "ushort", "using", "virtual", "void", "volatile", "while"};
plainToHtml = plainToHtml.Replace("\r\n", "<br />");
plainToHtml = plainToHtml.Replace(" ", "<span style='margin-left: 1em;' />");
string newKeyword = "";
foreach (string cSharpKeyword in cSharpKeywords)
{
newKeyword = @"\b" + cSharpKeyword + @"\b";
plainToHtml = Regex.Replace(plainToHtml, newKeyword, "<font style='color:#0000FF'>" + cSharpKeyword + "</font>");
}
return plainToHtml;
}